Laravel Queue System: A Complete Guide
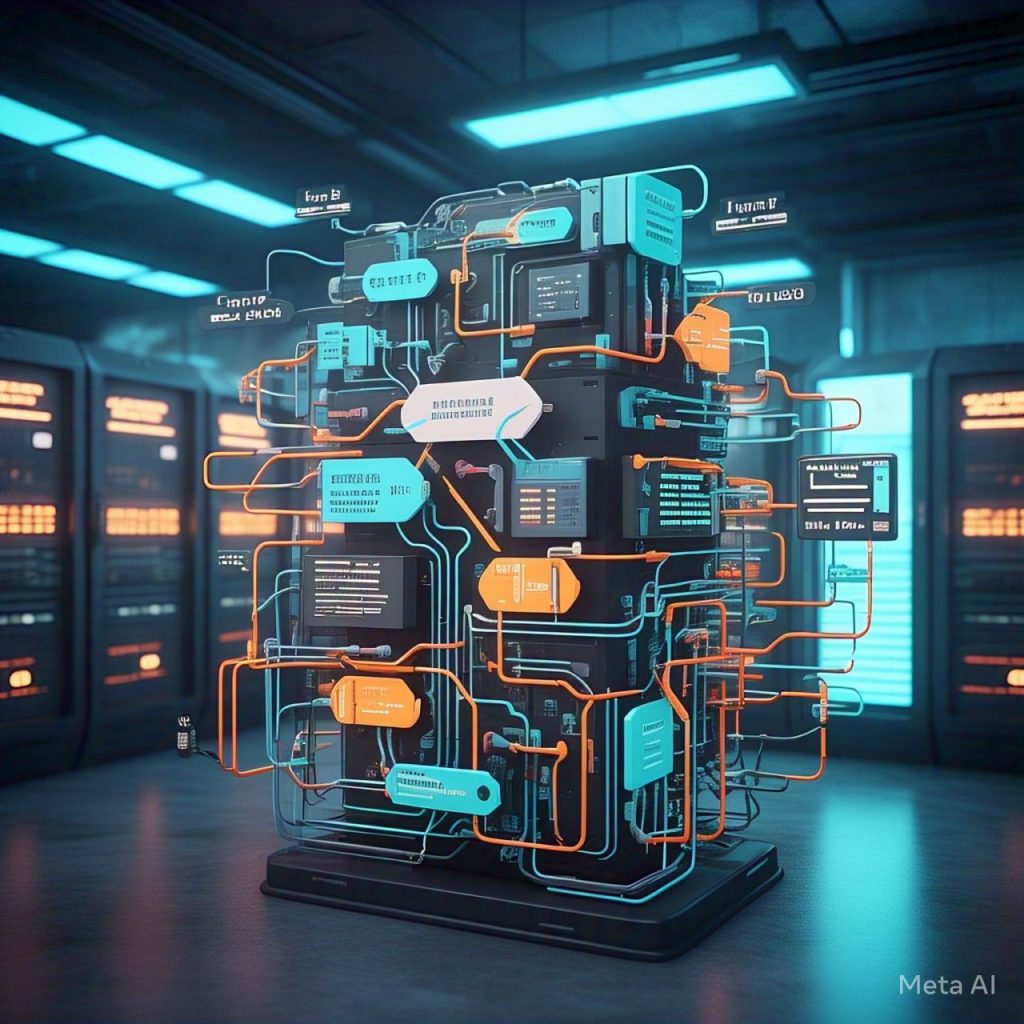
Laravel’s queue system allows you to handle long-running tasks in the background, improving your app’s performance and user experience. Instead of processing tasks immediately (which can slow down your app), Laravel queues push jobs into a queue and process them asynchronously.
Why Use Laravel Queues?
Improves Performance – Time-consuming tasks run in the background.
Prevents Request Timeouts – Users don’t have to wait for tasks to complete.
Scalable – Handles multiple jobs efficiently with queue workers.
Retries Failed Jobs – Ensures tasks are completed even after failures.
How Laravel Queues Work
- A job is dispatched (added to the queue).
- The job waits in the queue storage (e.g., database, Redis, SQS).
- A queue worker processes the job asynchronously.
- If a job fails, Laravel can retry it automatically.
Setting Up Laravel Queues (Step-by-Step)
Configure the Queue Driver
Laravel supports multiple queue drivers:
- sync – Processes jobs immediately (no queueing).
- database – Stores jobs in a database table.
- redis – Uses Redis for fast in-memory processing.
- sqs – Uses AWS Simple Queue Service (SQS).
Set the queue driver in your .env
file:
envCopyEditQUEUE_CONNECTION=database
For Redis:
envCopyEditQUEUE_CONNECTION=redis
Create a Job Class
Run the command:
bashCopyEditphp artisan make:job ProcessOrder
This creates a job in app/Jobs/ProcessOrder.php
. Open the file and modify the handle()
method:
phpCopyEditnamespace App\Jobs;
use App\Models\Order;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldBeUnique;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
class ProcessOrder implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $order;
public function __construct(Order $order)
{
$this->order = $order;
}
public function handle()
{
// Simulate processing an order
\Log::info('Processing order ID: ' . $this->order->id);
}
}
Dispatch the Job
Call this job in a controller or service:
phpCopyEdituse App\Jobs\ProcessOrder;
use App\Models\Order;
$order = Order::find(1);
ProcessOrder::dispatch($order);
Run the Queue Worker
Start processing queued jobs:
bashCopyEditphp artisan queue:work
For continuous processing, use:
bashCopyEditphp artisan queue:work --daemon
Handling Failed Jobs
If a job fails, Laravel retries it automatically. To manually retry failed jobs:
bashCopyEditphp artisan queue:retry all
To store failed jobs in the database, run:
bashCopyEditphp artisan queue:failed-table
php artisan migrate
Scaling Queues with Supervisor (For Production)
In production, you can use Supervisor to keep queue workers running:
- Install Supervisor: bashCopyEdit
sudo apt update && sudo apt install supervisor
- Create a configuration file for Laravel queues: bashCopyEdit
sudo nano /etc/supervisor/conf.d/laravel-worker.conf
Add this content: iniCopyEdit[program:laravel-worker] process_name=%(program_name)s_%(process_num)02d command=php /var/www/html/artisan queue:work --tries=3 autostart=true autorestart=true user=www-data numprocs=1 redirect_stderr=true stdout_logfile=/var/log/laravel-worker.log
- Reload Supervisor: bashCopyEdit
sudo supervisorctl reread sudo supervisorctl update sudo supervisorctl start laravel-worker:*
Conclusion
Laravel queues make background processing easy and efficient. Whether you’re sending emails, processing payments, or handling large tasks, queues ensure smooth app performance.
Are you using Laravel queues in your project? Let’s discuss your use case in the comments!